5.9. Parameters#
You can think of ROS parameters as node settings which are not hard-coded into the node’s code,
but which the node can query or update when it is just starting up
or even while the node or nodes are already running.
Parameters are best used to configure your robot.
For example, if you were building an autonomous vehicle and wanted to cap the maximum velocity of the vehicle at 100 km/h, you could create a parameter called max_speed
that is visible to all the nodes.
A node can store parameters as integers, floats, booleans, strings, and lists.
For more background on parameters, please see the ROS 2 Core Concepts section.
Note
In ROS 1, parameter values would be shared between nodes in the system (this is also called a “blackboard design pattern” in software engineering). However, in ROS 2 each node maintains its own set of parameters. This is one of the key design differences between ROS 1 and ROS 2.
Let’s take a look at the param
command by running ros2 param --help
.
$ ros2 param --help
Usage: ros2 param [-h] Call `ros2 param <command> -h` for more detailed usage. ...
Various param related sub-commands
options:
-h, --help show this help message and exit
Commands:
delete Delete parameter
describe Show descriptive information about declared parameters
dump Dump the parameters of a node to a yaml file
get Get parameter
list Output a list of available parameters
load Load parameter file for a node
set Set parameter
Call `ros2 param <command> -h` for more detailed usage.
At a high level, ROS 2’s param
command has sub commands to get and set a variable
along with a list
functionality and a delete
command.
As with most of the
other commands we’ve worked through, it is instructive to look at list
first.
5.9.1. Setup a demo ROS graph#
Before we can play with parameters, let’s make sure we have some nodes running.
If you have not done so already, start up the two turtlesim nodes, /turtlesim
and /teleop_turtle
.
Open a new terminal and run:
$ ros2 run turtlesim turtlesim_node
Open another terminal and run:
$ ros2 run turtlesim turtle_teleop_key
Note
As always, don’t forget to source your ROS 2 environment in every new terminal you open.
5.9.2. Listing available parameters#
Now let’s see what the docs say about the list
sub command and then see what
happens when we call the sub command.
Open a third terminal, and check the help on ros2 param list
:
$ ros2 param list --help
usage: ros2 param list [-h] [--spin-time SPIN_TIME] [-s] [--no-daemon]
[--filter FILTER] [--include-hidden-nodes]
[--param-prefixes PARAM_PREFIXES [PARAM_PREFIXES ...]]
[--param-type]
[node_name]
Output a list of available parameters
positional arguments:
node_name Name of the ROS node
options:
-h, --help show this help message and exit
--spin-time SPIN_TIME
Spin time in seconds to wait for discovery (only
applies when not using an already running daemon)
-s, --use-sim-time Enable ROS simulation time
--no-daemon Do not spawn nor use an already running daemon
--filter FILTER Only parameters matching the regex expression will be
showed. Supports `re` regex syntax.
--include-hidden-nodes
Consider hidden nodes as well
--param-prefixes PARAM_PREFIXES [PARAM_PREFIXES ...]
Only list parameters with the provided prefixes
--param-type Print parameter types with parameter names
The only possible argument of note in this sub command is the node_name
which allows
you to narrow the scope of param list
to only those parameters used by a particular
node.
So, to see the parameters belonging to your nodes, open a new terminal and enter the command:
$ ros2 param list
You will see the node namespaces, /teleop_turtle
and /turtlesim
, followed by each node’s parameters:
/teleop_turtle:
qos_overrides./parameter_events.publisher.depth
qos_overrides./parameter_events.publisher.durability
qos_overrides./parameter_events.publisher.history
qos_overrides./parameter_events.publisher.reliability
scale_angular
scale_linear
use_sim_time
/turtlesim:
background_b
background_g
background_r
qos_overrides./parameter_events.publisher.depth
qos_overrides./parameter_events.publisher.durability
qos_overrides./parameter_events.publisher.history
qos_overrides./parameter_events.publisher.reliability
use_sim_time
In terms of parameters in the turtlesim
node, we see that our call to param list
gives us background_x
(where x
is r
, g
or b
) and a use_sim_time
parameter.
Every node has the parameter use_sim_time
; it’s not unique to turtlesim.
Based on their names, it looks like /turtlesim
’s parameters determine the background color of the turtlesim window using RGB color values.
Note
There are also several qos_overrides.
parameters for each node, which can be set when starting the node to define its “Quality of Service” (QoS).
QoS can be used to tune the communication between nodes, based on the requirements of your application, e.g. to minimize communication latency or prioritize mission-critical information.
QoS is an advanced topic outside the scope of this manual.
To learn all about the param
command why don’t we try to change these background color parameters using the
CLI.
5.9.3. Getting parameters from the CLI#
The first step in changing the background color is to see what the current color is.
The param get
sub command requires both a node name and a parameter name.
In our list above we can see the node name as the top level element with
the forward slash in front of it, namely /turtlesim
.
The syntax for param get
is
$ ros2 param get <node_name> <parameter_name>
Let’s give it a whirl and see our current background color values.
First find the current value of /turtlesim
’s parameter background_b
:
$ ros2 param get /turtlesim background_b
Which will return the value:
Integer value is: 255
Now you know background_b
holds an integer value.
If you run the same command on background_r
and background_g
, you will get the values 69
and 86
, respectively.
On most computers color is represented as a triplet of <R,G,B>
values.
The color value of <69,86,255>
corresponds to a periwinkle blue color.
To change the color of the turtlesim we need to first set the parameter value and then reset the turtlesim to make it apply the color changes.
5.9.4. Setting parameters via the CLI#
To change a parameter’s value at runtime, use the command:
$ ros2 param set <node_name> <parameter_name> <value>
Let’s use this syntax to change /turtlesim
’s background color:
$ ros2 param set /turtlesim background_b 128
Your terminal should return the message:
Set parameter successful
And the background of your turtlesim window should change colors:
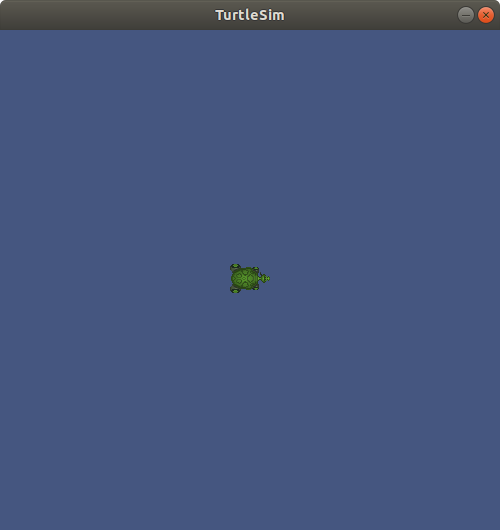
Setting parameters with the set
command will only change them in your current session, not permanently.
However, you can save your settings and reload them the next time you start a node.
5.9.5. Saving all parameters to a file#
When you stop a node, all its set parameter values will be lost. However, you can save your settings into a file and reload them the next time you start the node again.
You can view all of a node’s current parameter values by using the command:
$ ros2 param dump <node_name>
The command prints to the standard output (stdout
) by default but you can also redirect the parameter values into a file to save them for later.
To save your current configuration of /turtlesim
’s parameters into the file turtleparams.yaml
,
you can use basic unix shell syntax to redirect the stdout
to a file using the >
operator:
$ ros2 param dump /turtlesim > turtleparams.yaml
You will find a new file in the current working directory your shell is running in. If you open this file, you’ll see the following content:
/turtlesim:
ros__parameters:
background_b: 255
background_g: 86
background_r: 150
qos_overrides:
/parameter_events:
publisher:
depth: 1000
durability: volatile
history: keep_last
reliability: reliable
use_sim_time: false
Here, all parameter values are defined using YAML syntax. YAML is an easy and flexible format for computers to parse, but it is also easy for humans to read and write (more so than other common data formats like XML or JSON).
Dumping parameters comes in handy if you want to reload the node with the same parameters in the future.
5.9.6. Loading dumped parameters into a node#
Likewise, you can load parameters from a file to a currently running node using the command:
$ ros2 param load <node_name> <parameter_file>
Go to the first terminal where you started the turtlesim_node
and press CTRL+C
(or just click on the close button of the TurtleSim window) to stop the turtlesim.
Try getting the parameter again:
$ ros2 param get /turtlesim background_g
Node not found
As we can see, since there is no /turtlesim
node anymore,
its parameters are also gone.
Now, restart it again using the familiar ros2 run turtlesim turtlesim_node
.
You should again have a TurtleSim window, with the original background color.
Now try to load the turtleparams.yaml
file generated with ros2 param dump
into /turtlesim
node’s parameters, enter the command:
$ ros2 param load /turtlesim turtleparams.yaml
You should recover the custom background color, and your terminal will return the message:
Set parameter background_b successful
Set parameter background_g successful
Set parameter background_r successful
Set parameter qos_overrides./parameter_events.publisher.depth failed: parameter 'qos_overrides./parameter_events.publisher.depth' cannot be set because it is read-only
Set parameter qos_overrides./parameter_events.publisher.durability failed: parameter 'qos_overrides./parameter_events.publisher.durability' cannot be set because it is read-only
Set parameter qos_overrides./parameter_events.publisher.history failed: parameter 'qos_overrides./parameter_events.publisher.history' cannot be set because it is read-only
Set parameter qos_overrides./parameter_events.publisher.reliability failed: parameter 'qos_overrides./parameter_events.publisher.reliability' cannot be set because it is read-only
Set parameter use_sim_time successful
Note
Some parameters are “read-only”, and can only be modified at startup and not afterwards.
That is why there are some warnings for the qos_overrides
parameters in the output.
Since we understand the reason for the warnings and that it does not matter for this demo,
we can safely ignore them.
Exercise 5.1
Of course, you can also edit the turtleparams.yaml
file manually
to try out different values for red, green, and blue: just pick an integer between 0 and 255. After you saved the file, reload the file and see how the terminal background color changed.
Can you give our turtle a black background (i.e., set R
,G
and B
all to zero)?
5.9.7. Load parameter file on node startup#
To start the same node using your saved parameter values, use the syntax:
$ ros2 run <package_name> <executable_name> --ros-args --params-file <file_name>
This is the same command you always use to start turtlesim,
with the added command line options --ros-args
and --params-file
, followed by the file you want to load.
Note
Recall that the --ros-args
command line argument was explained earlier when we discussed remapping node names.
Stop your running turtlesim node (use CTRL+C
), and then try reloading it with your saved parameters, using:
$ ros2 run turtlesim turtlesim_node --ros-args --params-file turtleparams.yaml
The turtlesim window should appear as usual, but immediately with the alternative background color you set earlier.
Note
When a parameter file is used at node startup, all parameters (including the read-only ones) will be updated.
5.9.8. Setting individual parameters on node startup#
Parameter files are preferred when setting many or all parameters for a node.
However, it is also possible to specify just one or a few parameters for a new node directly using ros2 run
without a parameter file.
This can be convenient when debugging, or when the node does not require many custom parameter values.
The general syntax to set a parameter as part of a ros2 run
invocation is pretty similar to the one using the parameter file.
$ ros2 run <package_name> <executable_name> --ros-args -p <param_name>:=<param_value>
Note that the argument -p
is used to indicate that what will follow is a single parameter setting.
This argument must be repeated for every parameter you set.
Also notice the :=
(not =
and no spaces) between the parameter name and value,
which is also similar to the syntax for remapping node names.
For example, to start another turtlesim_node
with just a red background,
we would set the background_g
and background_b
parameters to zero:
$ ros2 run turtlesim turtlesim_node --ros-args -p background_g:=0 -p background_b:=0
The newly created window should have only a dark red background.
5.9.9. Summary#
Nodes have parameters to define their default configuration values.
You can get
and set
parameter values from the command line.
You can also save the parameter settings to a file to reload them in a future session.