6.1. Launch files#
Up until this point we’ve been running single ROS programs by hand using the run
command,
and you had to open new terminals for every new node you run.
As you create more complex systems with more and more nodes running simultaneously, opening terminals and reentering configuration details becomes tedious. Indeed, this is not how larger ROS systems are generally operated, and needing to run each node separately and with the right configuration parameters is extremely error prone. Many robots require to start up tens if not hundreds of small nodes!
This is where the ros2 launch
command comes in:
it can be used to execute ROS launch files that execute multiple programs in one go.
The launch system in ROS 2 is responsible for helping the user describe the configuration of their system and then execute it as described. The configuration of the system includes what programs to run, where to run them, what arguments to pass them, and ROS-specific conventions which make it easy to reuse components throughout the system by giving them each a different configuration. It is also responsible for monitoring the state of the processes launched, and reporting and/or reacting to changes in the state of those processes.
To define, run and configure a number of ROS 2 nodes that should be started simultaneously,
a description should be provided to ros2 launch
.
Such descriptions are called launch files.
In ROS 2, launch files can be written in Python, XML, or YAML,
and can start and stop different nodes as well as trigger and act on various events.
Launch files can also be used to set parameters when launching nodes,
or remap names and topics for key components of your robotics system.
Note
Launch files are usually included with a ROS package and are commonly stored in a
launch
subdirectory.
Note that launch files written in Python typically use the .launch.py
file extension,
those in XML use the .launch.xml
file extension,
and those in YAML use the .launch.yml
file extension.
If properly setup, running a single launch file with the ros2 launch
command will start up your entire system - all nodes and their configurations - at once.
6.1.1. Launch from the CLI#
The ROS launch command is different from most of the other ROS commands in that it has no sub commands and has a single function. To illustrate this command let’s take a look at its help file.
$ ros2 launch -h
usage: ros2 launch [-h] [-d] [-p | -s] [-a]
package_name [launch_file_name]
[launch_arguments [launch_arguments ...]]
Run a launch file
positional arguments:
package_name Name of the ROS package which contains the launch file
launch_file_name Name of the launch file
launch_arguments Arguments to the launch file; '<name>:=<value>' (for
duplicates, last one wins)
optional arguments:
-h, --help show this help message and exit
-d, --debug Put the launch system in debug mode, provides more
verbose output.
-p, --print, --print-description
Print the launch description to the console without
launching it.
-s, --show-args, --show-arguments
Show arguments that may be given to the launch file.
-a, --show-all-subprocesses-output
Show all launched subprocesses' output by overriding
their output configuration using the
OVERRIDE_LAUNCH_PROCESS_OUTPUT envvar.
The launch
command has two arguments,
the first one is the package name and then the launch file name.
Tip
If you are unaware of the launch files in your package you can use TAB-completion after ros2 launch
to list all the available launch files!
Finally, some launch files have arguments that can be
appended to the command. If you are unsure about what a launch file does, or
what arguments it needs, the --print
and --show-args
commands will tell you
this information.
Note
You already knew that packages can contain multiple executables. Now you can see that packages can also be used to collect zero or more launch files. Sometimes developers create a package containing no executables and only launch files to startup their robot’s main processes!
6.1.2. Example#
The turtlesim
package contains also a launch file, multisym.launch.py
.
Let’s read up on the multisym.launch.py
launch file and then
run it following the example below (end the simulation with CTRL-C
):
$ ros2 launch turtlesim multisim.launch.py --show-args
Arguments (pass arguments as '<name>:=<value>'):
No arguments.
This shows the launch file does not require any (optional) arguments.
To see what launching this launch file would do, use --print
:
$ ros2 launch turtlesim multisim.launch.py --print
<launch.launch_description.LaunchDescription object at 0x7f75aab63828>
├── ExecuteProcess(cmd=[ExecInPkg(pkg='turtlesim', exec='turtlesim_node'), '--ros-args'], cwd=None, env=None, shell=False)
└── ExecuteProcess(cmd=[ExecInPkg(pkg='turtlesim', exec='turtlesim_node'), '--ros-args'], cwd=None, env=None, shell=False)
From this description we can deduce that this launch file would execute two processes, both from the same package (turtlesim
) and both the same executable (turtlesim_node
).
Let’s “launch” the launch file!
$ ros2 launch turtlesim multisim.launch.py
[INFO] [launch]: All log files can be found below /home/kscottz/.ros/log/2020-06-24-14-39-03-312667-kscottz-ratnest-20933
[INFO] [launch]: Default logging verbosity is set to INFO
[INFO] [turtlesim_node-1]: process started with pid [20944]
[INFO] [turtlesim_node-2]: process started with pid [20945]
^C[WARNING] [launch]: user interrupted with ctrl-c (SIGINT)
This should run two turtlesim nodes:
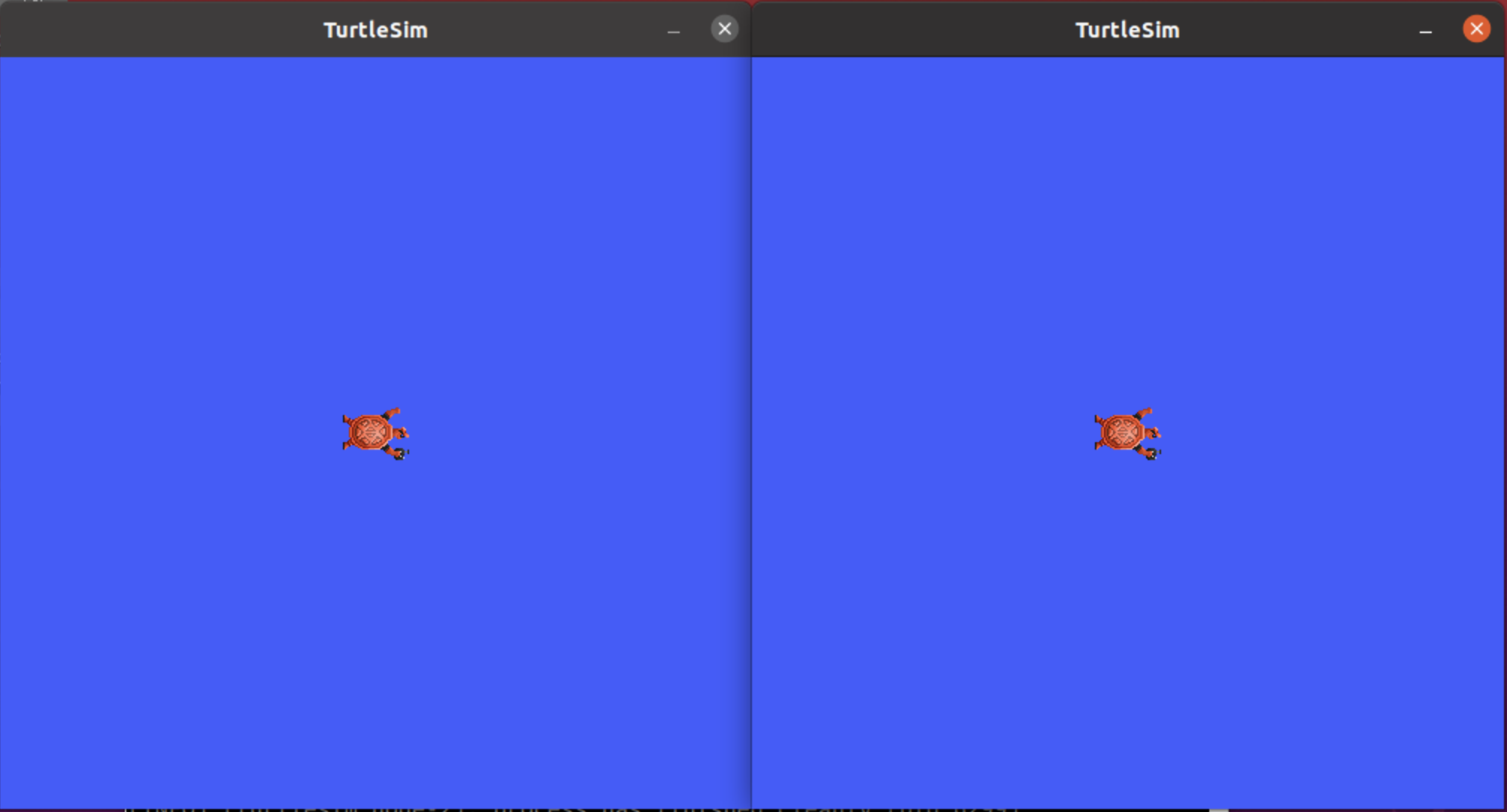
Exercise 6.1
Try stopping the ros2 launch
process that you just started by pressing CTRL-C in the terminal.
What happens to the two turtlesim nodes that were started by ros2 launch
, are they still running or have they been stopped as well?
How can you use this property to easily stop complex ROS graphs consisting of many nodes?
To continue the instructions below, relaunch the launch file after this exercise.
6.1.3. Python, XML, and YAML for ROS 2 Launch Files#
ROS 2 launch files can be written in Python, XML, and YAML.
As you may have guessed from the .py
file extension, multisim.launch.py
was a Python file.
And indeed, if you would have located the launch file on your file system and inspected it,
you would see it is a simple Python file:
# turtlesim/launch/multisim.launch.py
from launch import LaunchDescription
import launch_ros.actions
def generate_launch_description():
return LaunchDescription([
launch_ros.actions.Node(
namespace= "turtlesim1", package='turtlesim', executable='turtlesim_node', output='screen'),
launch_ros.actions.Node(
namespace= "turtlesim2", package='turtlesim', executable='turtlesim_node', output='screen'),
])
For now, don’t worry about the contents of this launch file, the Python code simply generates a description of what executables to run in what packages.
In case you do not need Python for complex program logic, one can also use XML and YAML to define the startup instructions of a launch file. You can see a comparison of these formats in the ROS 2 documentation on launch formats. You can find more information on ROS 2 launch in the online ROS 2 launch tutorials.
6.1.3.1. (Optional) Control the Turtlesim Nodes#
Now that these nodes are running, you can control them like any other ROS 2 nodes. For example, you can make the turtles drive in opposite directions by opening up two additional terminals and running the following commands:
In the second terminal:
ros2 topic pub /turtlesim1/turtle1/cmd_vel geometry_msgs/msg/Twist "{linear: {x: 2.0, y: 0.0, z: 0.0}, angular: {x: 0.0, y: 0.0, z: 1.8}}"
In the third terminal:
ros2 topic pub /turtlesim2/turtle1/cmd_vel geometry_msgs/msg/Twist "{linear: {x: 2.0, y: 0.0, z: 0.0}, angular: {x: 0.0, y: 0.0, z: -1.8}}"
After running these commands, you should see something like the following:
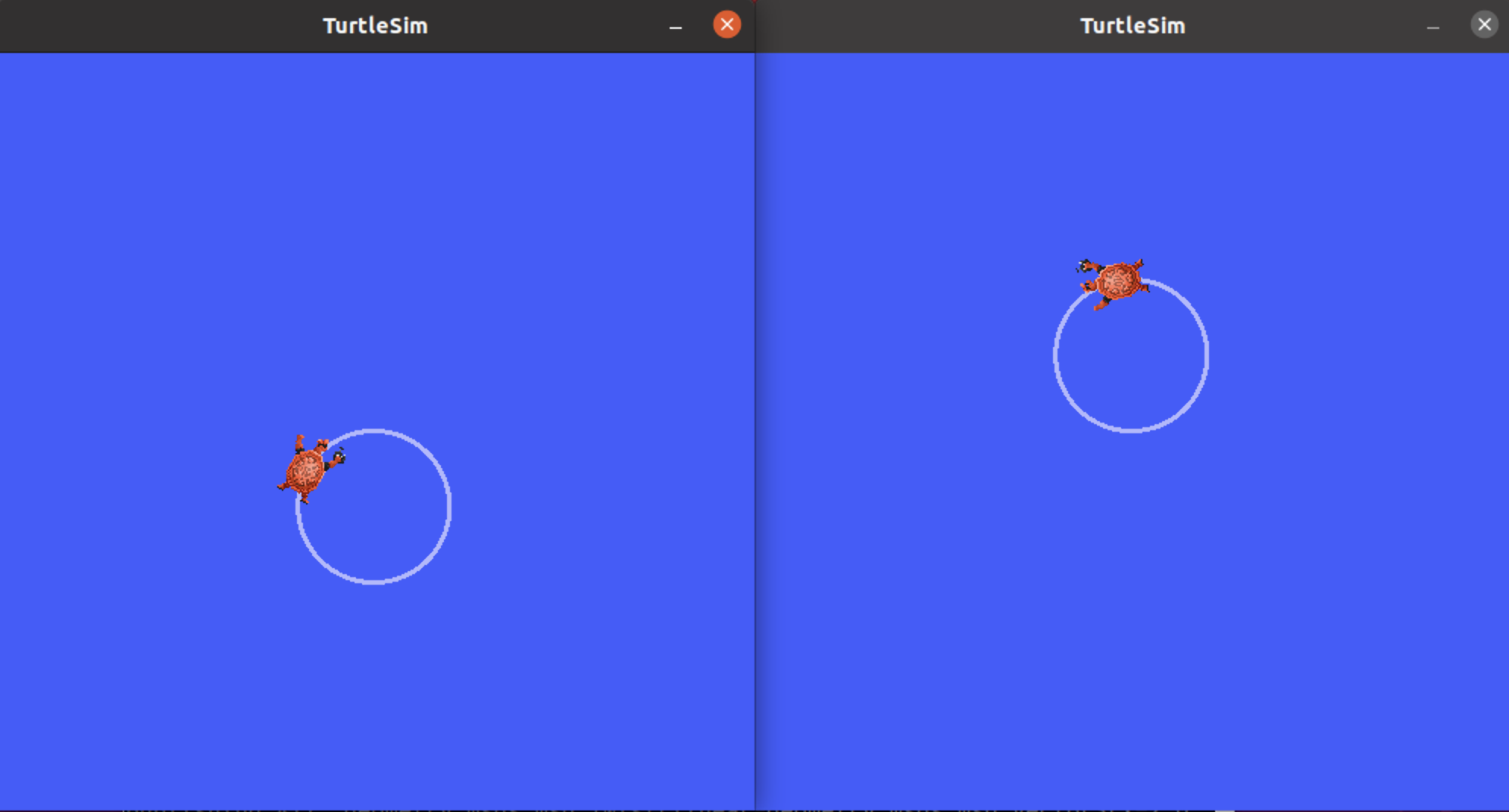
6.1.4. Summary#
The significance of what you’ve done so far is that you’ve run two turtlesim nodes with one command.
Once you learn to write your own launch files, you’ll be able to run multiple nodes - and set up their configuration - in a similar way, with the ros2 launch
command.
For more tutorials on ROS 2 launch files, see the online ROS 2 launch tutorials.